Documentation
Ctrl+K
Image Cropper
Image Cropper is an easy to use jQuery plugin for image cropping with support of live previews and custom aspect ratio. For more details
Round Crop
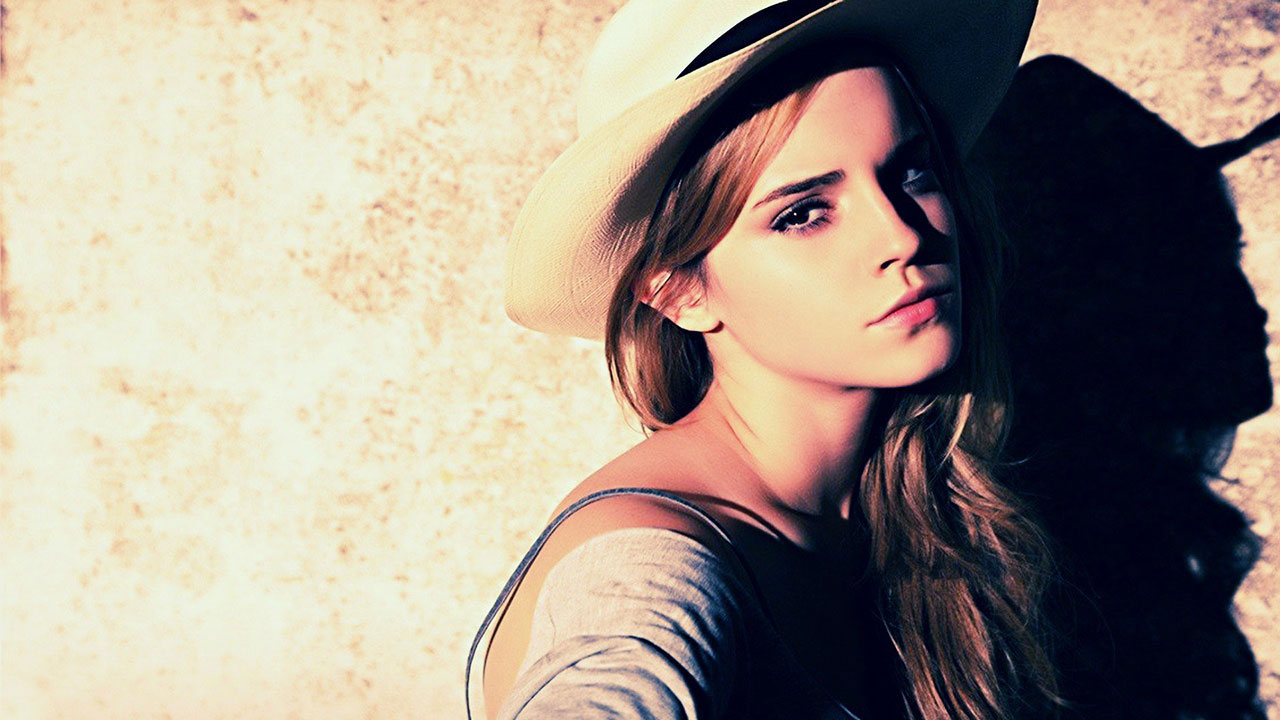
Preview
HTML Code
<div class="card">
<div class="card-body">
<div class="row">
<div class="col-md-6 text-center">
<div class="croppy-round">
<img src="../assets/images/plugins/01.jpg" alt="picture" class="img-fluid" id="round-crop" loading="lazy">
</div>
<button id="roundButton" class="btn btn-primary my-2">Crop</button>
</div>
<div class="col-md-6 text-center">
<h5>Preview</h5>
<div id="roundResult"></div>
</div>
</div>
</div>
</div>
Script
window.onload = function () {
function getRoundedCanvas(sourceCanvas) {
const roundcanvas = document.createElement('canvas');
const context = roundcanvas.getContext('2d');
let width = sourceCanvas.width;
let height = sourceCanvas.height;
roundcanvas.width = width;
roundcanvas.height = height;
context.imageSmoothingEnabled = true;
context.drawImage(sourceCanvas, 0, 0, width, height);
context.globalCompositeOperation = 'destination-in';
context.beginPath();
context.arc(width / 2, height / 2, Math.min(width, height) / 2, 0, 2 * Math.PI, true);
context.fill();
return roundcanvas;
}
let roundImage = document.getElementById('round-crop');
let roundButton = document.getElementById('roundButton');
let roundResult = document.getElementById('roundResult');
let roundcroppable = false;
let roundCropper = new Cropper(roundImage, {
aspectRatio: 1,
viewMode: 1,
ready: function () {
roundcroppable = true;
},
});
roundButton.onclick = function () {
let croppedCanvas;
let roundedCanvas;
let roundedImage;
if (!roundcroppable) {
return;
}
// Crop
croppedCanvas = roundCropper.getCroppedCanvas();
// Round
roundedCanvas = getRoundedCanvas(croppedCanvas);
// Show
roundedImage = document.createElement('img');
roundedImage.src = roundedCanvas.toDataURL()
roundedImage.classList = "img-fluid w-50";
roundResult.innerHTML = '';
roundResult.appendChild(roundedImage);
};
};